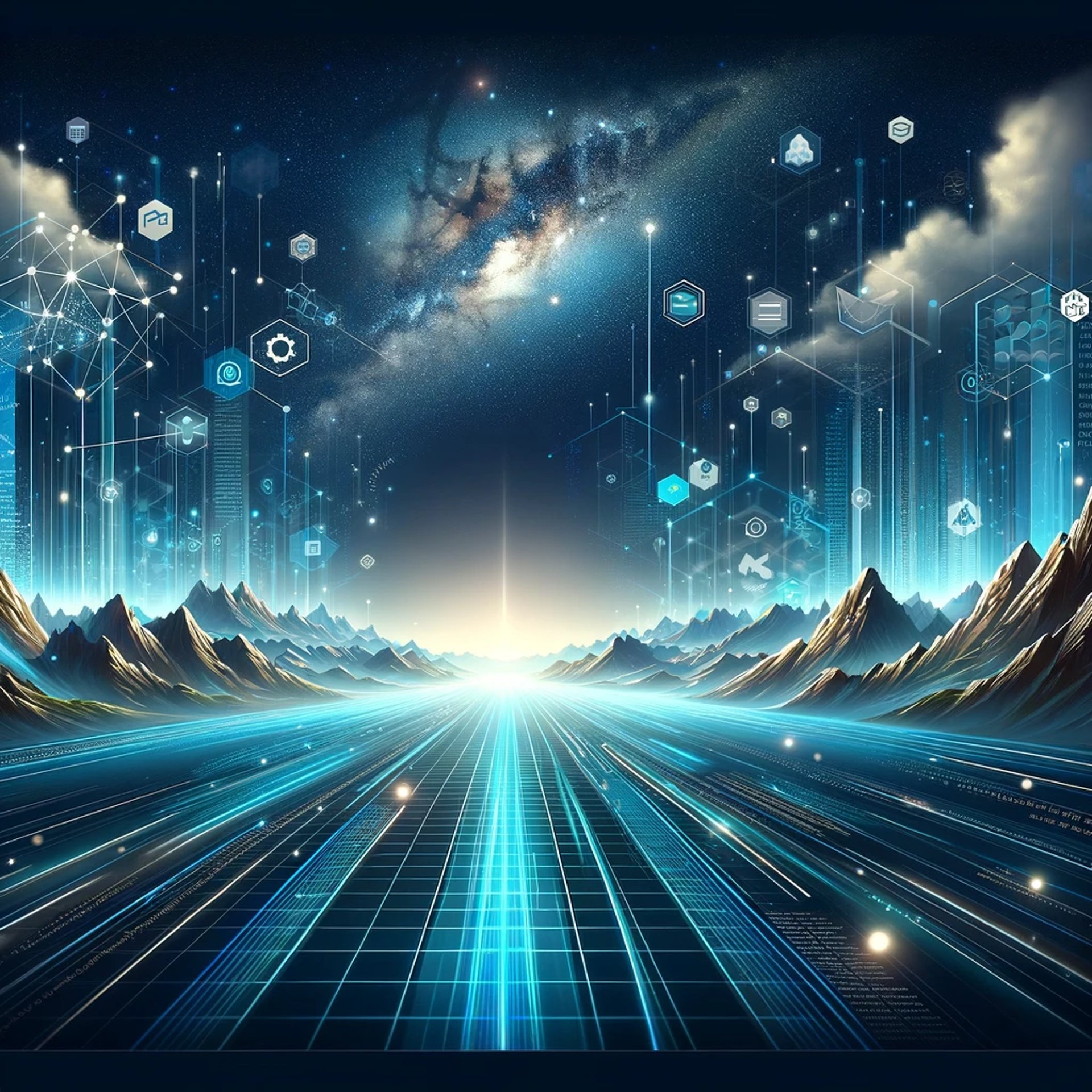
Salesforce Best Practice: Using Unit of Work
Learn how to implement the Unit of Work pattern in Salesforce Apex to maintain clean, organized, and scalable code. Discover the benefits of using the Unit of Work class for ensuring Separation of Concerns and simplifying complex transactions.
SALESFORCE BEST PRACTICES
8/11/20243 min read
In Salesforce Apex development, managing complex transactions across multiple objects while maintaining clean and organized code can be challenging. The Unit of Work pattern offers a robust solution to this problem, ensuring that your code adheres to the Separation of Concerns (SoC) principle. In this blog post, we’ll explore what the Unit of Work pattern is, why it’s important, and how to structure and implement it effectively in your Salesforce projects.
What Is the Unit of Work Pattern?
The Unit of Work pattern is a design pattern that helps manage changes to multiple objects during a transaction in a way that ensures consistency and reduces the risk of errors. It acts as a central point for managing DML operations (inserts, updates, deletes) and allows for the coordination of these operations across different parts of your application. By encapsulating these operations within a Unit of Work class, you can commit all changes at once, ensuring that your code is more maintainable, testable, and adheres to the SoC principle.
Why Use the Unit of Work Pattern?
1. Consistency and Atomicity
The Unit of Work pattern ensures that all changes within a transaction are consistent. If one operation fails, none of the changes are committed, preventing partial updates that could lead to data inconsistencies.
2. Simplified Transaction Management
By centralizing DML operations within the Unit of Work class, you reduce the complexity of managing transactions across multiple objects, making your code easier to read and maintain.
3. Enhanced Testability
Isolating DML operations in a Unit of Work class allows for easier mocking during testing, enabling you to test business logic without worrying about actual database operations.
How to Structure a Unit of Work Class
1. Naming Conventions
The Unit of Work class should be named to reflect its purpose clearly. For instance, you might name it UnitOfWork or TransactionManager.
2. Methods in the Unit of Work Class
The Unit of Work class should contain methods that handle registering and committing DML operations. These methods should allow for adding records to be inserted, updated, or deleted and then committing these changes as a single transaction.
Example Structure:
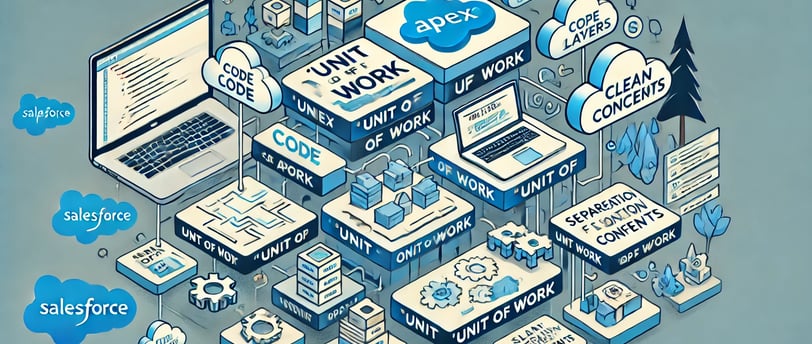
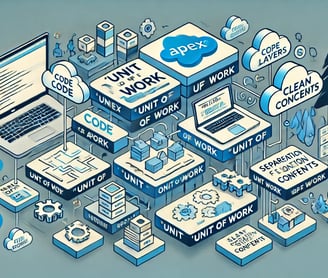
3. Usage in Service Classes
Service classes should use the Unit of Work class to manage transactions, ensuring that all related DML operations are handled together. This keeps the service classes focused on business logic while delegating transaction management to the Unit of Work class.
Example Usage in a Service Class:
4. Handling Complex Scenarios
For more complex scenarios involving multiple related objects, the Unit of Work class can be extended to manage different types of records and their relationships, ensuring that all operations are committed together.
Example of a Complex Unit of Work:
Best Practices for Unit of Work Classes
Centralize DML Operations: Ensure all DML operations are managed through the Unit of Work class to maintain consistency and simplify transaction management.
Focus on Business Logic: Let service classes handle business logic while the Unit of Work class manages transactions, keeping your code modular and maintainable.
Document Methods: Clearly document the methods within your Unit of Work class to make the code easier to understand and maintain.
Combine with Other Patterns: Use the Unit of Work class in conjunction with service and selector classes to create a well-structured and scalable application.
Conclusion
The Unit of Work pattern is a powerful tool in Salesforce Apex development that promotes clean, organized, and maintainable code by ensuring that all changes within a transaction are managed consistently. By implementing a Unit of Work class, you can adhere to the Separation of Concerns principle, making your application more scalable, testable, and easier to maintain. Incorporate the Unit of Work pattern into your development practices to handle complex transactions with confidence and precision.
FAQs
1. What is the Unit of Work pattern in Salesforce Apex? The Unit of Work pattern manages changes to multiple objects during a transaction, ensuring consistency and simplifying complex operations by centralizing DML operations.
2. How does the Unit of Work pattern improve code maintainability? By centralizing DML operations in a Unit of Work class, it reduces complexity, making the code easier to maintain, update, and test.
3. Should the Unit of Work class handle business logic? No, the Unit of Work class should focus solely on managing transactions. Business logic should be handled by service classes.
4. Can the Unit of Work pattern be used with other design patterns? Yes, the Unit of Work pattern is often used in conjunction with service and selector classes to create a well-structured and scalable application.
5. How does the Unit of Work pattern enhance testability in Salesforce? By isolating DML operations in a Unit of Work class, it becomes easier to mock these operations during unit tests, allowing for more accurate and efficient testing.
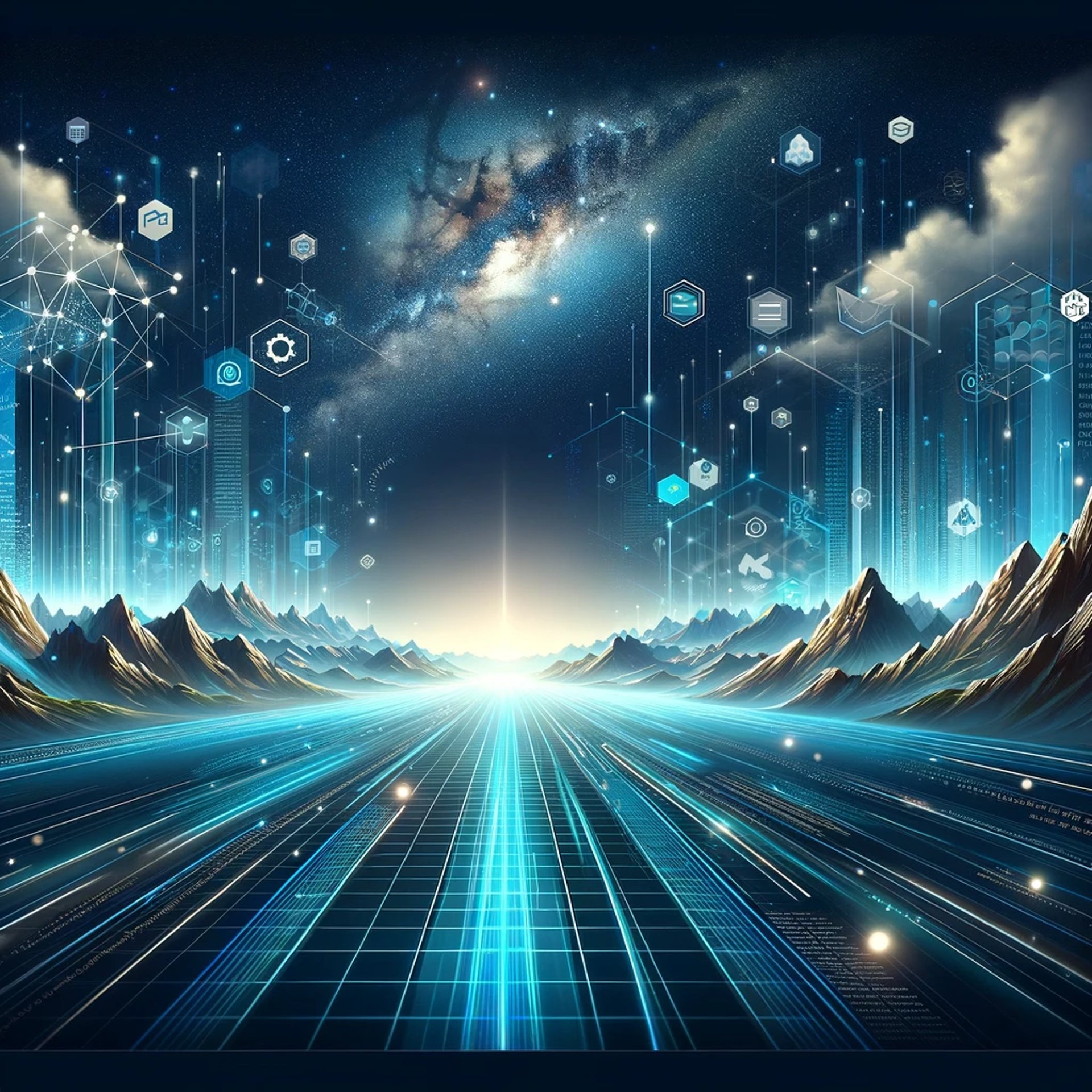