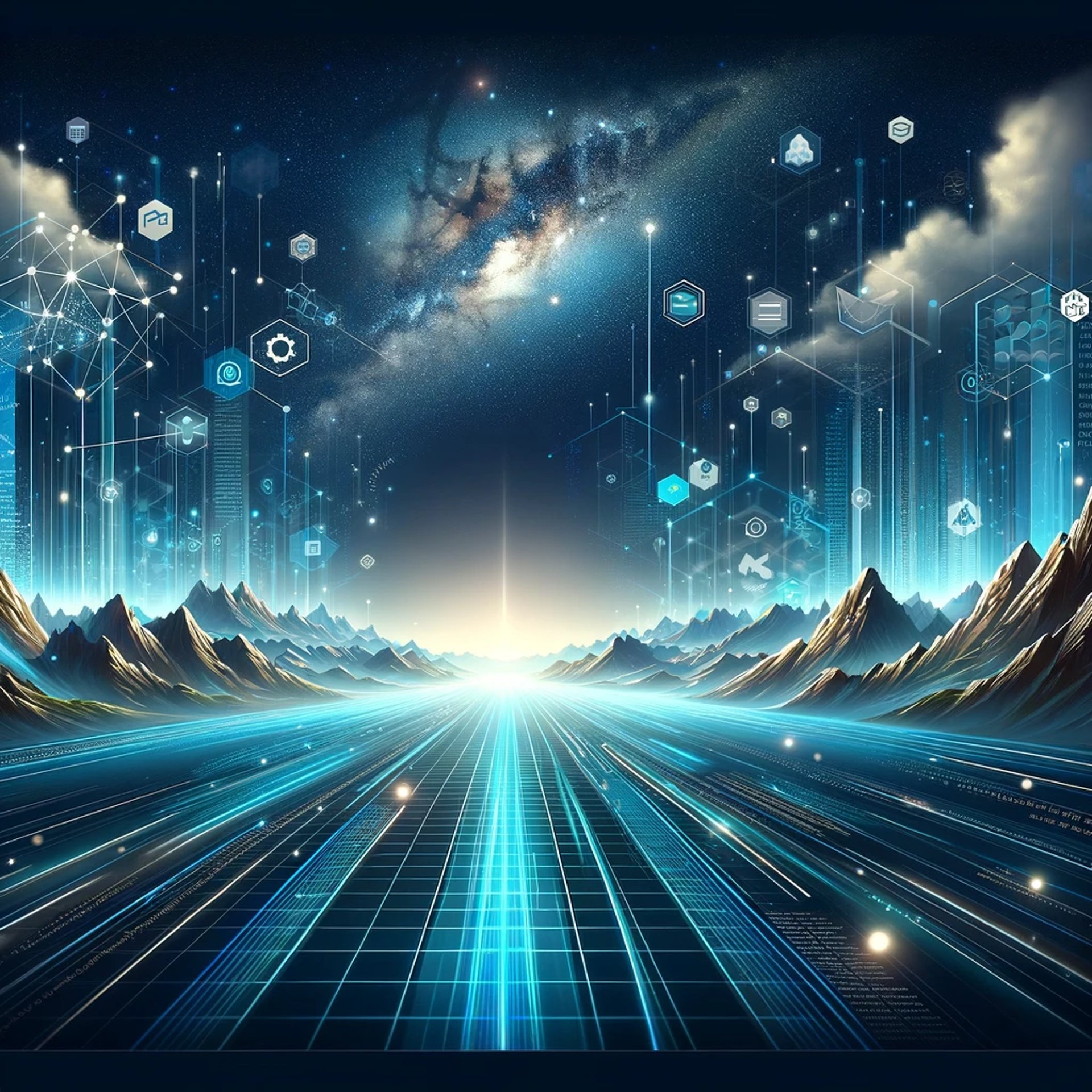
Salesforce Best Practice: Using @TestVisible in Apex
Discover how to use the @TestVisible annotation in Salesforce Apex to improve testability without compromising code encapsulation. Learn best practices for making private methods and variables accessible in your unit tests.
SALESFORCE BEST PRACTICES
8/20/20243 min read
In Salesforce Apex development, writing comprehensive unit tests is essential for ensuring the reliability and quality of your code. However, private methods and variables—critical to encapsulation and code organization—can sometimes be challenging to test directly. This is where the @TestVisible annotation comes into play. In this blog post, we’ll explore what @TestVisible is, how to use it effectively, and where it’s most useful in your Salesforce Apex code.
What Is @TestVisible?
@TestVisible is an annotation in Salesforce Apex that allows you to make private variables and methods visible to your test classes. This annotation provides a way to maintain the encapsulation of your code while still enabling you to write thorough unit tests that validate the internal workings of your classes.
Example of @TestVisible:
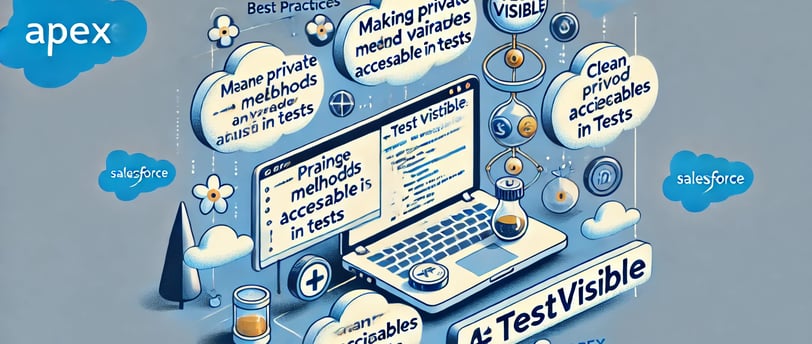
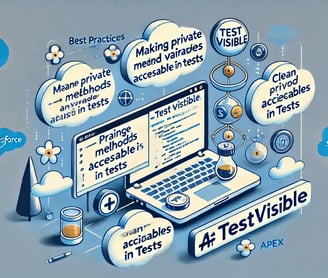
In this example, DEFAULT_DISCOUNT and applyDiscount are private members of the AccountService class, but they are marked with @TestVisible, making them accessible for testing.
Why Use @TestVisible?
1. Improve Test Coverage
Using @TestVisible allows you to write tests for private methods and variables, ensuring that every part of your logic is covered. This leads to higher code coverage and more reliable tests.
2. Maintain Encapsulation
One of the key principles of object-oriented programming is encapsulation—keeping internal details of a class hidden from the outside world. @TestVisible lets you preserve encapsulation in your production code while allowing your tests to access necessary details.
3. Ensure Code Quality
By making private members testable, you can verify the correctness of your logic at a granular level. This helps catch bugs early and ensures that your code behaves as expected in all scenarios.
How to Use @TestVisible Effectively
1. Marking Private Variables and Methods
Use @TestVisible to mark private variables and methods that you need to access in your unit tests. This is especially useful for logic that is critical to the functioning of your class but doesn’t need to be exposed to other classes.
2. Writing Unit Tests for Private Members
Once a variable or method is marked with @TestVisible, you can access it directly in your test classes to validate its behavior.
Example Test Class:
3. Avoid Overuse
While @TestVisible is powerful, it should be used judiciously. Only mark members as @TestVisible when it’s absolutely necessary for testing. Overusing this annotation can lead to a situation where too many internal details are exposed, reducing the effectiveness of encapsulation.
4. Combine with Dependency Injection
To reduce the need for @TestVisible, consider using dependency injection or other design patterns that naturally make your code more testable. This keeps your code clean and your tests comprehensive without relying too heavily on the annotation.
Where Is @TestVisible Most Useful?
1. Complex Business Logic
In classes with complex business logic, @TestVisible allows you to test internal calculations, validations, and decision-making processes that are encapsulated within private methods.
2. Static Variables
When you have static variables that control important aspects of your class behavior, marking them with @TestVisible allows you to verify their values and ensure they are set correctly.
3. Helper Methods
Helper methods that are private but perform critical operations can be made testable using @TestVisible, ensuring that even the smallest parts of your logic are validated.
Conclusion
The @TestVisible annotation is an essential tool for Salesforce Apex developers who want to maintain code encapsulation while ensuring comprehensive test coverage. By making private methods and variables accessible in tests, @TestVisible allows you to validate every aspect of your logic without compromising the integrity of your code. Incorporate @TestVisible into your development practices to write robust, maintainable, and well-tested Salesforce applications.
FAQs
1. What is @TestVisible in Salesforce Apex? @TestVisible is an annotation that allows private variables and methods to be accessible in test classes, improving test coverage without compromising code encapsulation.
2. Why should I use @TestVisible? Using @TestVisible helps you write comprehensive tests that validate the internal logic of your classes, ensuring higher code quality and reliability.
3. How do I use @TestVisible in my code? You can apply the @TestVisible annotation to private methods and variables that you need to test, making them accessible in your unit tests.
4. Should I use @TestVisible on all private members? No, use @TestVisible sparingly, only when necessary for testing. Overusing it can reduce the effectiveness of encapsulation.
5. Can @TestVisible be combined with other testing best practices? Yes, combine @TestVisible with design patterns like dependency injection to reduce the need for the annotation while still ensuring testability.
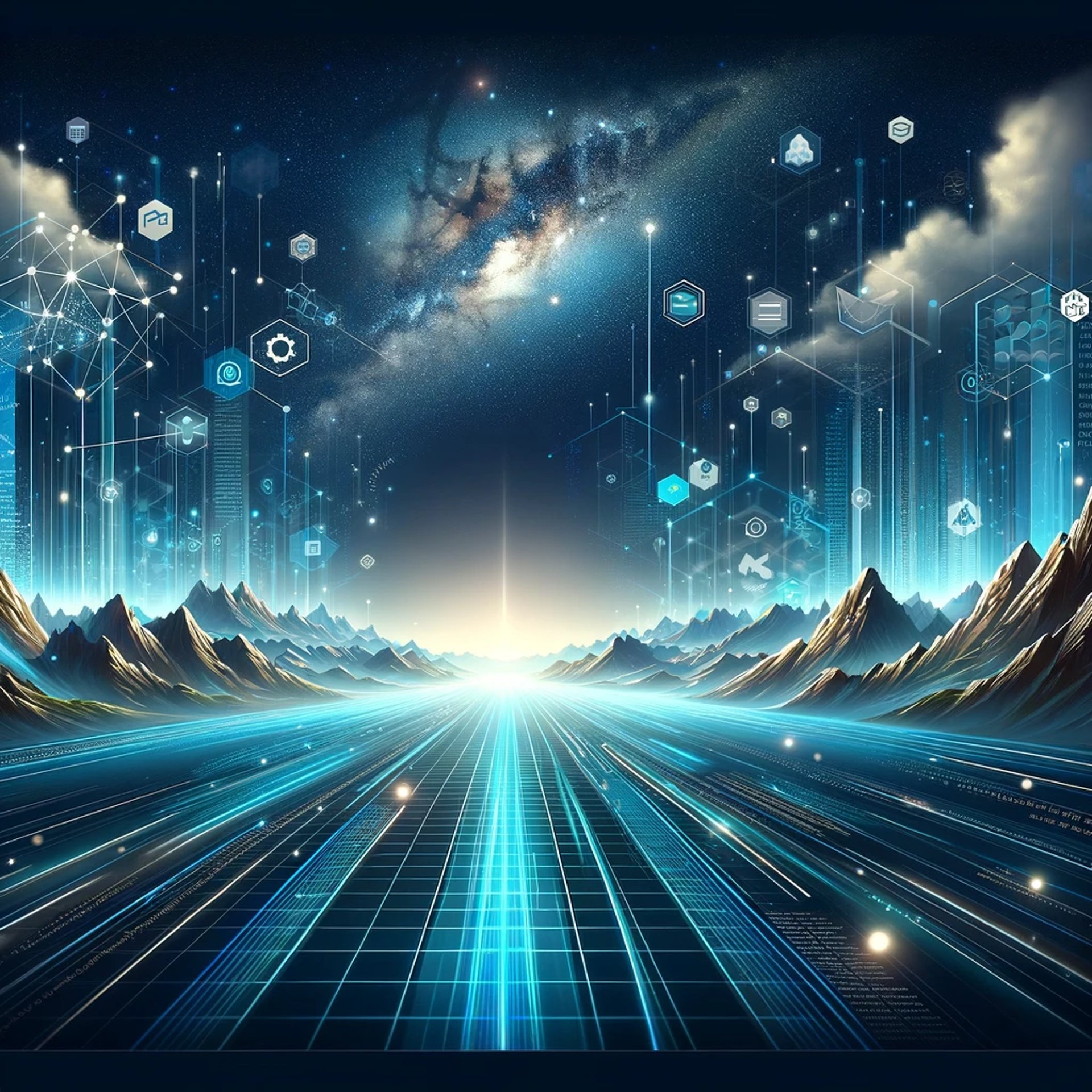