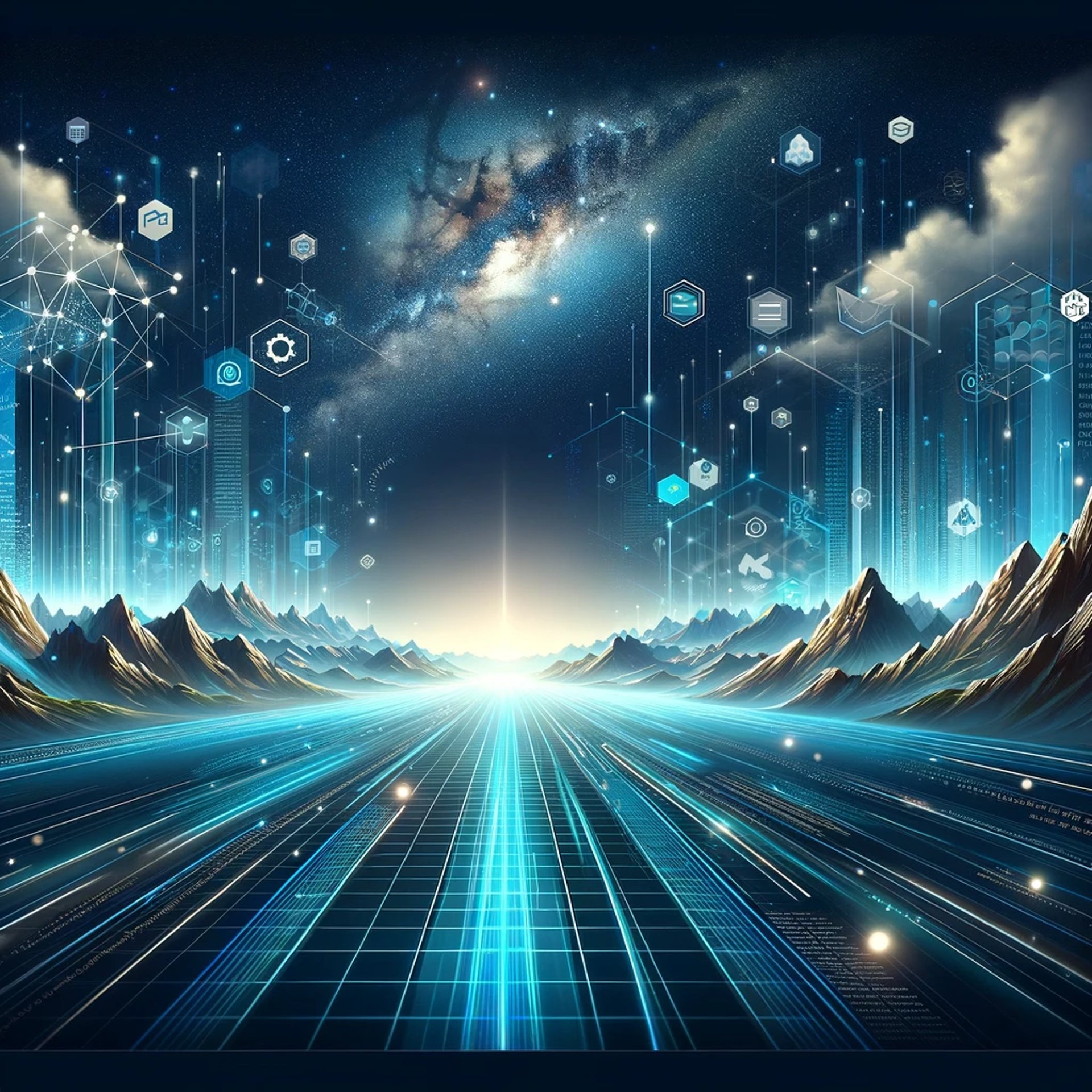
Salesforce Best Practice: Using Constants in Salesforce Apex
Discover best practices for using constants in Salesforce Apex to improve code clarity, maintainability, and testability. Learn how to handle magic numbers and strings, name constants based on their meaning, and determine the right visibility for constants in your Apex classes.
SALESFORCE BEST PRACTICES
8/7/20242 min read
In the world of Salesforce Apex, using constants effectively can transform your code from a tangled mess into a clear, maintainable, and robust system. Let's dive into some best practices for handling constants, focusing on magic numbers, strings, naming conventions, and visibility.
Magic Numbers Should Be Constants
Ever stumbled upon a number in your code and wondered what it represents? Those are magic numbers. The term "magic number" refers to numeric literals that have an unclear meaning or purpose. Making them constants helps in several ways:
Clarity: It becomes obvious what the number represents.
Maintainability: Changing the value is easier as you only need to do it in one place.
Testing: Constants can be referenced in tests, reducing the need to keep values in sync.
For instance:
@TestVisible private static final Integer MAX_LENGTH_NAME = 80;
@TestVisible private static final Integer NUM_RECORDS_PER_PAGE = 20;
@TestVisible private static final Integer MAX_RECORDS = 10;
Strings Should Be Constants (Except Empty Strings)
Using constants for strings helps avoid typos, standardizes references, and simplifies changes. Instead of repeatedly typing out the same string value, you define it once and use the constant everywhere.
Example:
public static final String ACCOUNT_RECORD_TYPE_CUSTOMER = 'Customer';
@TestVisible private static final String ERROR_PRODUCT_QUANTITY_ZERO = 'Product must have a Quantity greater than 0.';
This approach minimizes errors and makes updates straightforward since changing the constant value updates it everywhere in the code.
Constants Should Be Named Based on Meaning, Not Value
Names should reflect the constant's purpose rather than its current value. For example, although OPPORTUNITY_STAGENAME_NEW and CASE_STATUS_NEW might both be "New", their meanings differ. Avoid using a constant in multiple contexts just because the values happen to match. Future changes to these values could otherwise introduce bugs.
Visibility of Constants: @TestVisible and Private
In most cases, constants should be @TestVisible and private:
@TestVisible private static final Integer MAX_NAME_LENGTH = 80;
However, there are exceptions. If a constant is used across a small, related set of classes, it might be defined as public:
public static final String ORDER_TYPE_SERVICE = 'Service';
This provides flexibility while maintaining encapsulation.
Conclusion
In Salesforce Apex, leveraging constants effectively can significantly enhance your code's readability, maintainability, and robustness. By making magic numbers and strings constants, naming them meaningfully, and carefully considering their visibility, you create a more professional and error-resistant codebase.
FAQs
1. Why should magic numbers be converted to constants? Magic numbers lack clear meaning. Converting them to constants enhances code readability and makes updates easier.
2. When should strings be constants in Apex? Strings should be constants to avoid typos, create standard references, and simplify changes, except for empty strings.
3. How should constants be named in Apex? Name constants based on their meaning, not their value, to avoid confusion and potential bugs from future changes.
4. Where should widely-used constants be placed? Place widely-used constants in a centralized Constants class, while class-specific constants should remain within their respective classes.
5. What visibility should Apex constants have? Generally, constants should be @TestVisible and private, but may be public if used by a small set of related classes.
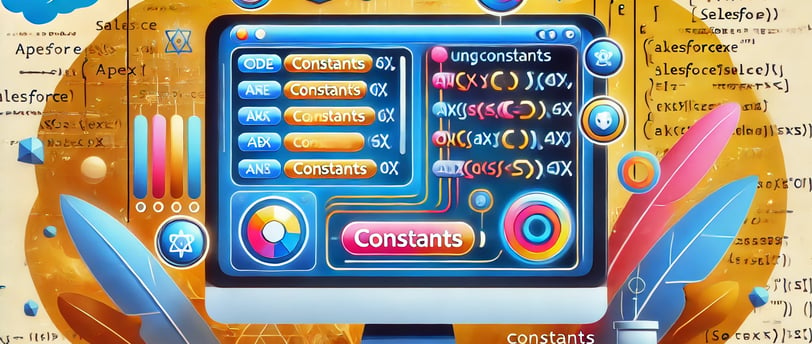
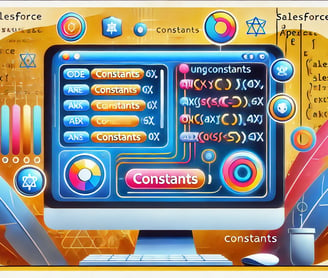
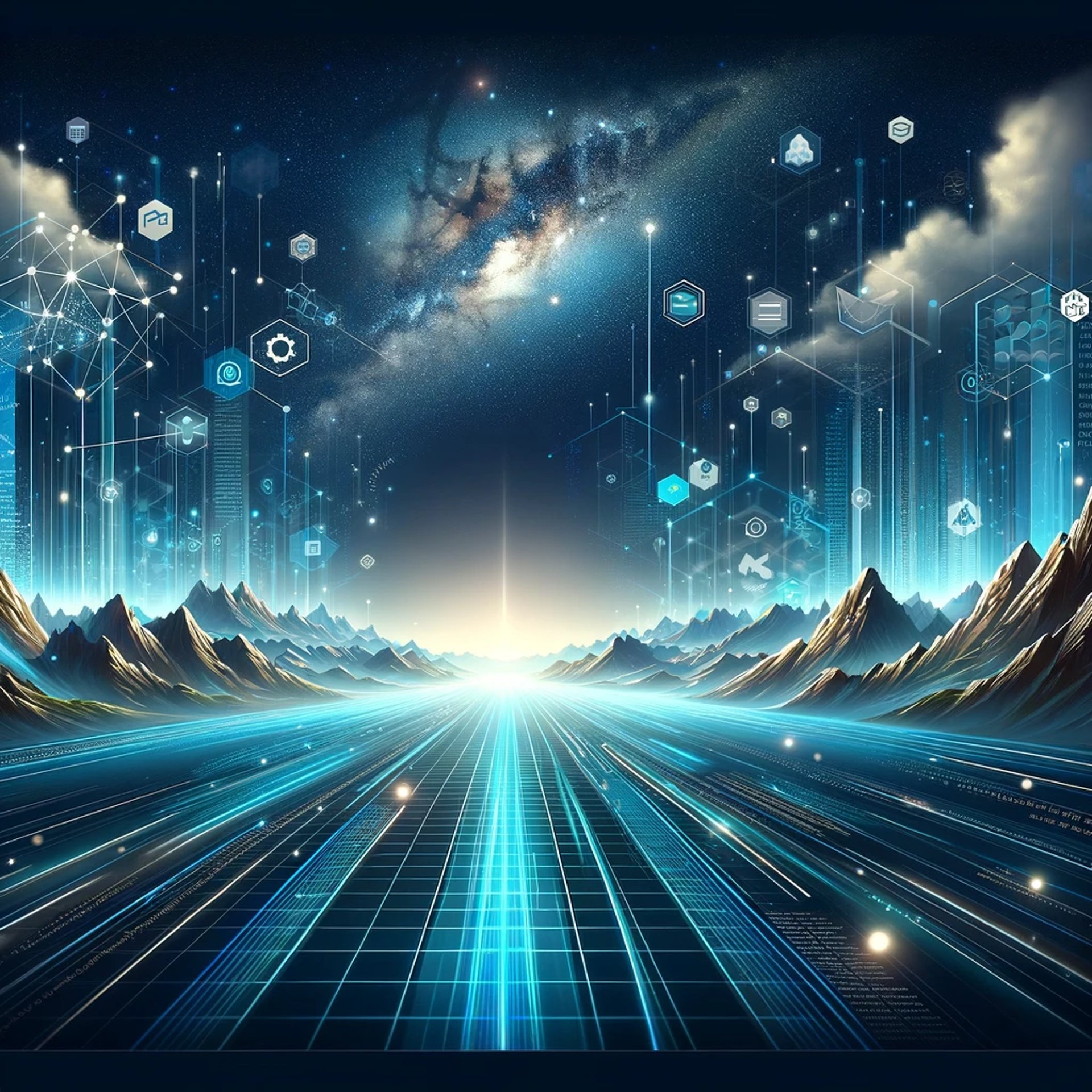