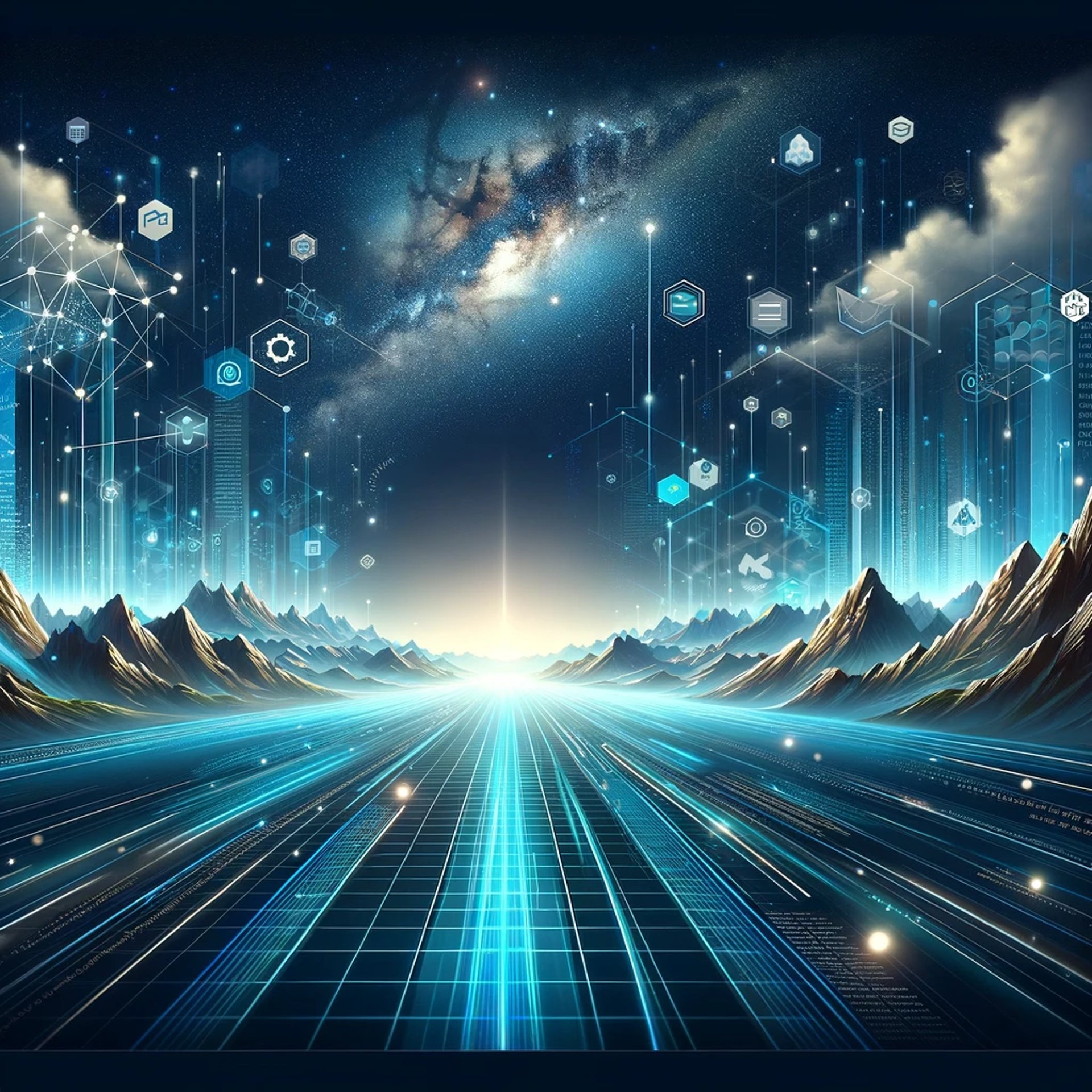
Salesforce Best Practice: How to Handle "Attempt to De-reference a Null Object" Error
Learn how to troubleshoot and avoid the "Attempt to de-reference a null object" error in Salesforce Apex. Discover best practices to prevent null pointer exceptions and ensure your code runs smoothly.
SALESFORCE BEST PRACTICES
8/29/20241 min read
One of the most common and frustrating errors encountered in Salesforce Apex development is the "Attempt to de-reference a null object" error. This error occurs when your code tries to access or modify an object that hasn’t been initialized, leading to a null pointer exception. In this blog post, we’ll dive into what causes this error, how to troubleshoot it, and best practices to avoid encountering it in the future.
Understanding the "Attempt to De-reference a Null Object" Error
In Salesforce Apex, a null pointer exception occurs when your code attempts to use an object that hasn't been assigned a value or has been explicitly set to null. This error is typically thrown when you try to access a property or method of a null object, leading to a breakdown in your code's logic.
Common Scenarios That Cause Null Pointer Exceptions:
Uninitialized Objects: Forgetting to initialize an object before using it.
Null Returned from a Method: A method returns null when you expect an object.
Conditional Logic Errors: Failing to check if an object is null before accessing its properties or methods.
Example of the Error:
Account acc; System.debug(acc.Name); // This line will throw a null pointer exception because 'acc' is null.
In this example, the Account object acc is not initialized, and attempting to access its Name property causes a null pointer exception.
How to Troubleshoot the Error
1. Identify the Source of the Null Object
The first step in troubleshooting is to identify where the null object is being referenced in your code. This typically involves reviewing the stack trace provided in the error message to pinpoint the line where the exception occurred.
2. Check Object Initialization
Ensure that the object is properly initialized before being used. If an object is expected to be populated from a database query or an API call, verify that the query or call successfully returns a result.
Example:
Account acc = [SELECT Id, Name FROM Account WHERE Id = :accountId LIMIT 1]; if (acc != null) { System.debug(acc.Name); } else { System.debug('No account found with the specified ID.'); }
3. Use Conditional Statements
Before accessing properties or methods of an object, use conditional statements to check if the object is null. This prevents your code from attempting to de-reference a null object.
Example:
if (acc != null && acc.Name != null) { System.debug(acc.Name); } else { System.debug('Account or Account Name is null.'); }
4. Implement the Safe Navigation Operator
Salesforce Apex provides the Safe Navigation Operator (?.), which allows you to safely navigate through an object’s properties. If the object is null, the expression returns null instead of throwing an exception.
Example:
String accountName = acc?.Name; // If 'acc' is null, 'accountName' will be null instead of causing an error.
Best Practices to Avoid Null Pointer Exceptions
1. Always Initialize Objects
Whenever you declare an object, ensure it is initialized before use. This can be done by assigning a default value or by using a constructor.
Example:
Account acc = new Account();
2. Validate Data from External Sources
When your code depends on data from external sources, such as database queries, APIs, or user input, always validate the data before processing it. Ensure that the data meets your expectations and handle cases where it does not.
3. Use Try-Catch Blocks
For scenarios where null pointer exceptions might be unavoidable, use try-catch blocks to gracefully handle the error and provide meaningful feedback.
Example:
try { System.debug(acc.Name); } catch (NullPointerException e) { System.debug('NullPointerException caught: ' + e.getMessage()); }
4. Document Assumptions
When writing code, document any assumptions about the initialization and state of objects. This helps others (and your future self) understand the conditions under which the code operates and can prevent errors from creeping in during maintenance.
Conclusion
The "Attempt to de-reference a null object" error is a common but preventable issue in Salesforce Apex development. By understanding the root causes, applying best practices for object initialization and validation, and using tools like the Safe Navigation Operator, you can avoid null pointer exceptions and ensure that your code runs smoothly. Take the time to implement these strategies in your Salesforce development process to reduce errors and improve the reliability of your applications.
FAQs
1. What is the "Attempt to de-reference a null object" error in Salesforce? This error occurs when your code tries to access or modify a property or method of an object that is null, leading to a null pointer exception.
2. How can I troubleshoot a null pointer exception in Salesforce? Identify the source of the null object, check object initialization, use conditional statements, and consider implementing the Safe Navigation Operator to avoid this error.
3. What is the Safe Navigation Operator in Salesforce? The Safe Navigation Operator (?.) allows you to safely access an object’s properties without throwing an exception if the object is null.
4. How can I prevent null pointer exceptions in my code? Always initialize objects, validate data from external sources, use try-catch blocks, and document assumptions to prevent null pointer exceptions.
5. Why is it important to avoid null pointer exceptions? Avoiding null pointer exceptions is crucial for ensuring that your code runs smoothly, improving its reliability, and providing a better user experience.
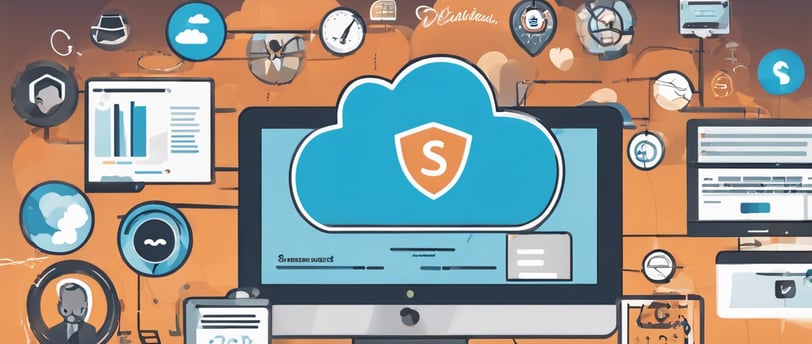
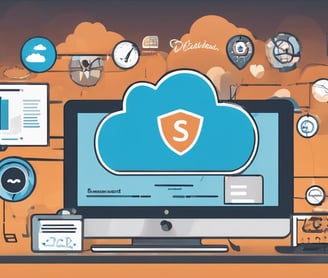
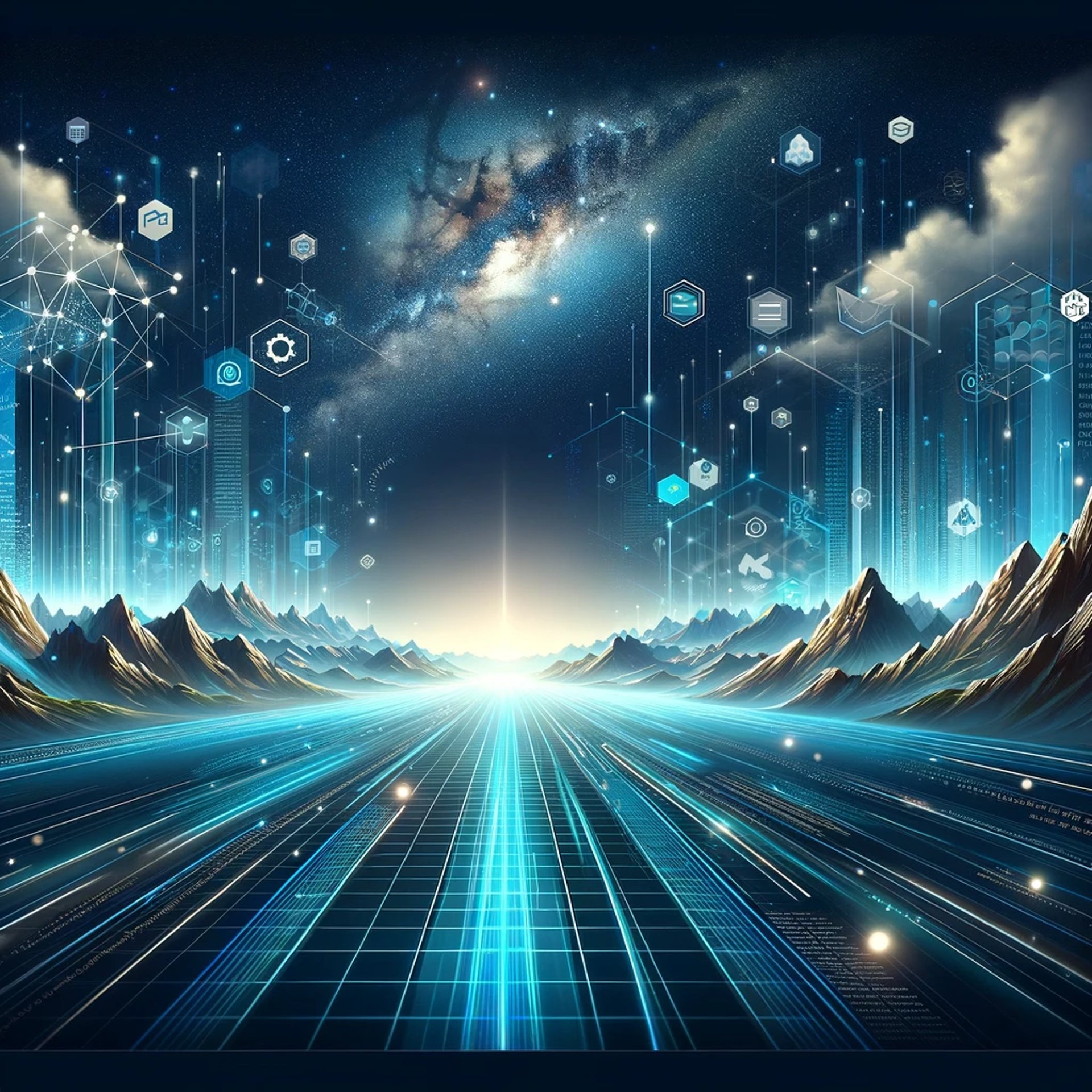