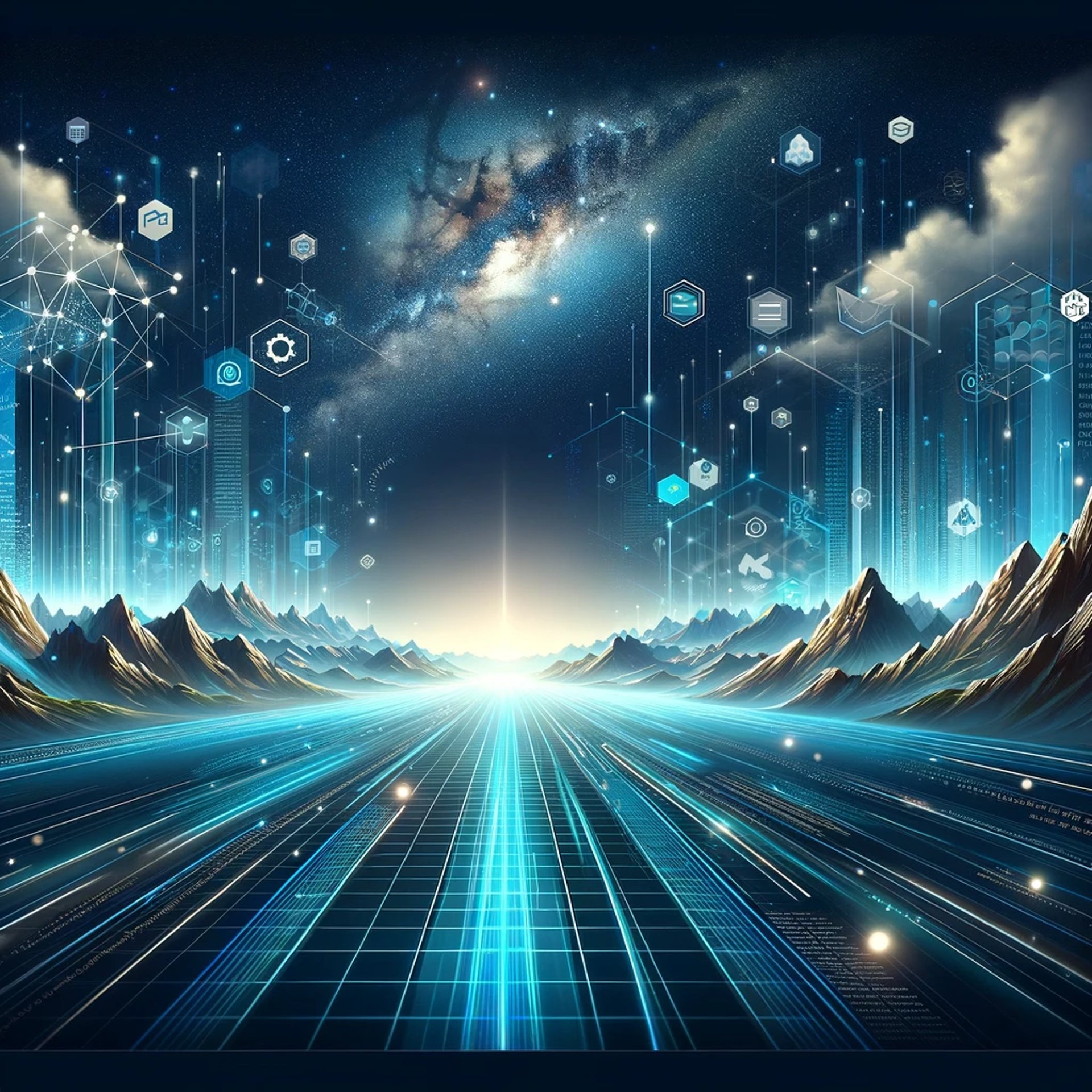
Salesforce Best Practice: How to Avoid the "List Has No Rows for Assignment to SObject" Error
Learn how to avoid the "List has no rows for assignment to SObject" error in Salesforce. Follow these best practices to prevent empty query results and improve error handling in your Apex code.
SALESFORCE BEST PRACTICES
9/16/20242 min read
In Salesforce development, one common error developers face is the "List has no rows for assignment to SObject" error. This occurs when a SOQL query returns no records, but the result is directly assigned to a single SObject variable. In this post, we’ll walk you through best practices to prevent this error and demonstrate how the null coalescing operator (??) can help handle empty query results.
Understanding the "List Has No Rows for Assignment to SObject" Error
This error occurs when a SOQL query returns no results, but your code expects at least one record, assigning it to a single SObject variable. If no records are found, Salesforce throws the error because there's nothing to assign.
Error Example: Account acc = [SELECT Id, Name FROM Account WHERE Name = 'NonExistentAccount'];
When no record matches the query, Salesforce throws the "List has no rows for assignment to SObject" error.
Best Practices to Avoid the Error
1. Use the Null Coalescing Operator (??)
The null coalescing operator (??) allows you to assign a default value when the left-hand query result is null. This ensures that your code will never throw the error, as it will always have a fallback SObject.
Example:
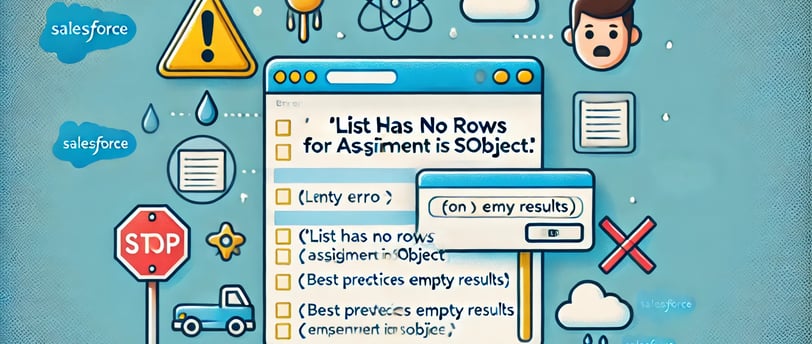
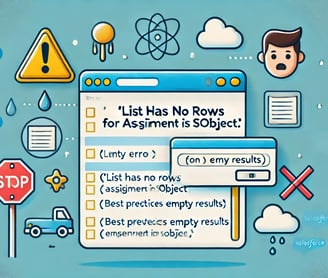
Here, if no account is found, it defaults to an empty Account() object.
2. Query into a List
Instead of directly assigning query results to an SObject, query into a list and check if any results exist before accessing them. This prevents the "no rows" error.
Example:
3. Use Try-Catch Blocks for SOQL Queries
By wrapping your query inside a try-catch block, you can handle the QueryException that occurs when no records are found, avoiding disruption to your code execution.
Example:
4. Use LIMIT in Queries
Using the LIMIT clause ensures your query only returns the required number of results, making it more efficient and avoiding any unnecessary resource consumption.
Example:
Conclusion
The "List has no rows for assignment to SObject" error is a common pitfall in Salesforce development, but it can be easily avoided with a few best practices. By using the null coalescing operator (??), querying into lists, and handling errors with try-catch blocks, you can prevent this error and ensure your Apex code runs smoothly.
FAQs
1. What causes the "List has no rows for assignment to SObject" error? The error occurs when a SOQL query doesn’t return any records, but the result is assigned directly to a single SObject variable.
2. How do I prevent this error in my code? Use the null coalescing operator (??), query into a list, or use a try-catch block to handle empty query results.
3. Why is using LIMIT important in SOQL queries? LIMIT restricts the number of records returned by your query, making it more efficient and preventing unnecessary resource consumption.
4. What is the null coalescing operator in Apex? The null coalescing operator (??) allows you to assign a default value if the left-hand side of an expression returns null. It's useful for avoiding null-related errors.
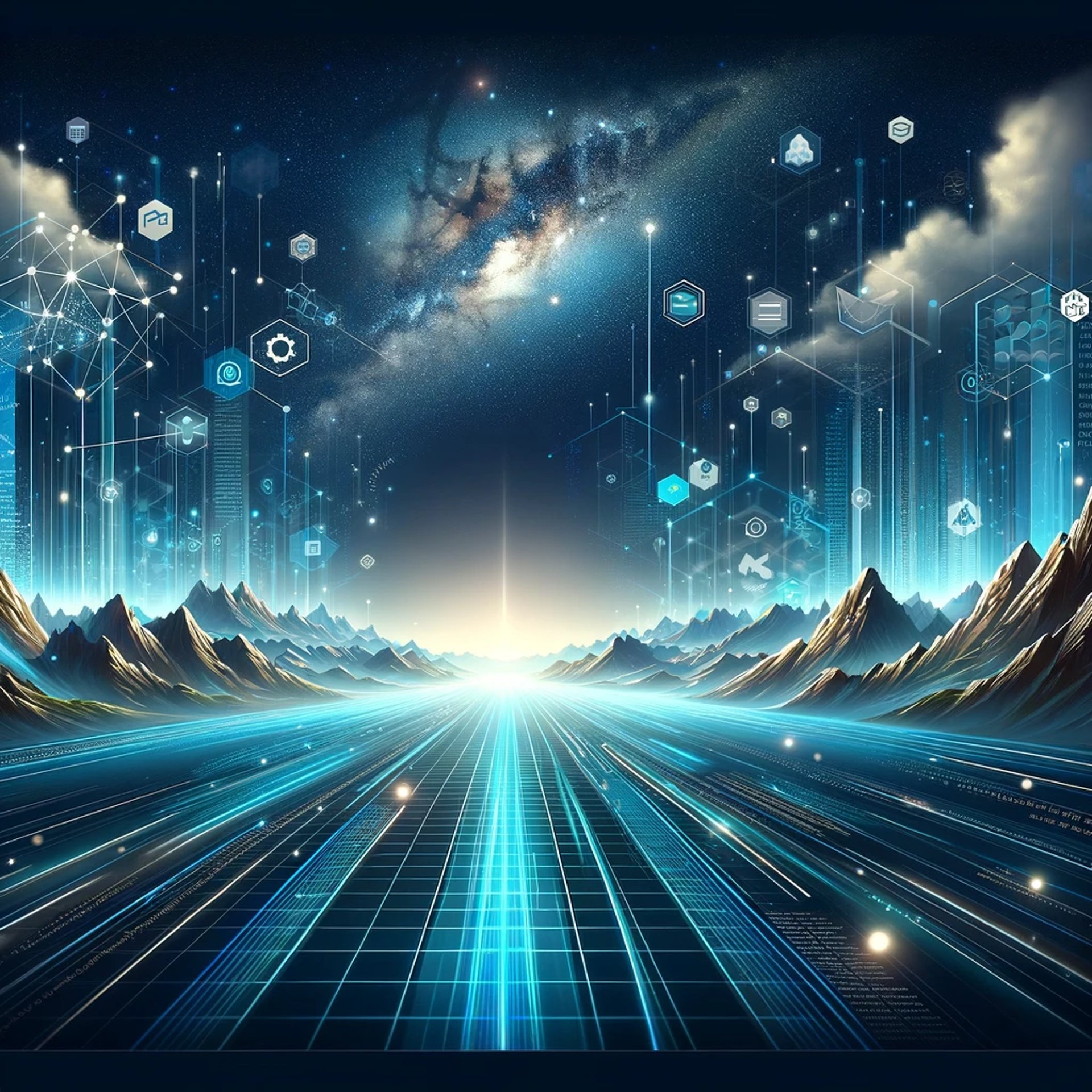